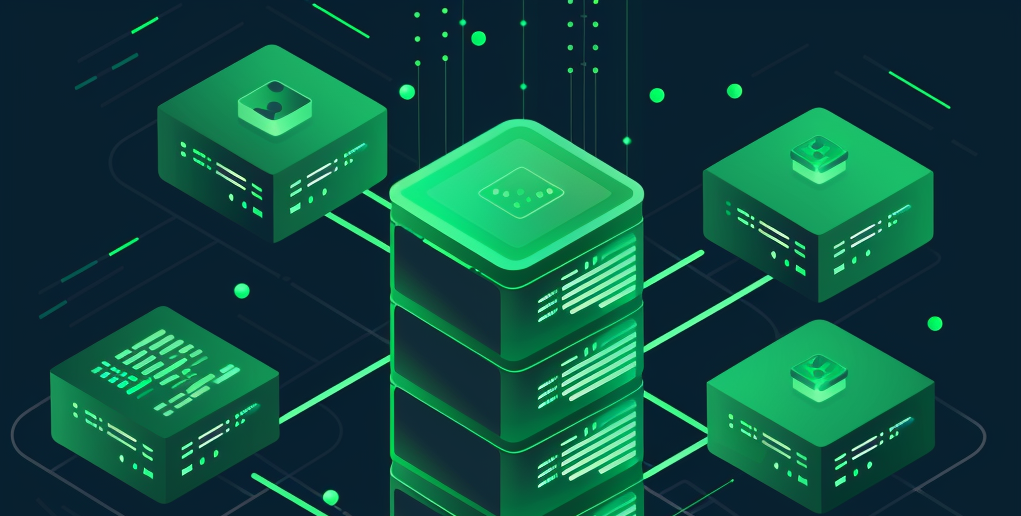
How to test Inertia.js partial reloads
Tim Geisendörfer • March 31, 2024
laravel inertia testingHey guys several months after my last blog post, so it was finally time to write a new one. I hope you will enjoy it.
In this blog post, we will explore how to test Inertia.js partial reloads in Laravel applications. I will try to cover how the partial reloads work and how you can test them in your Laravel applications.
For further information about this feature you can check the official Inertia.js documentation.
Why you should use Inertia.js partial reloads
Inertia.js partial reloads are a powerful feature that allows you to update specific parts of your page without having
to refresh the entire page. This can be useful in a variety of scenarios, such as when you want to update a chat window,
a list of messages, or a form without losing the current state of the page.
It can also be handy when you only need to load data after the page initially was rendered using the Inertia::lazy()
method. Usually you want to do that for ressource intensive data which is not needed on the first page load.
How Inertia.js partial reloads work
The Inertia.js frontend library handles partial reloads by sending an XHR request to the server with the necessary information to reload a specific component or data. The server then responds with the updated data, which the frontend library uses to update the page without refreshing it.
Inertia under the hood uses the X-Inertia-Partial-Component
and X-Inertia-Partial-Data
headers to identify partial
reloads in the request. The X-Inertia-Partial-Component
header tells inertia which component is the origin for the
request, and the X-Inertia-Partial-Data
header tells Inertia which data should be reloaded.
How to use Inertia.js partial reloads
You need to structure your Laravel Controller in a way that it can handle partial reloads. This means that you need to return your data in closures. Here is an example of how you can structure your controller:
class ChatController extends Controller
{
public function show()
{
return Inertia::render('Chat/Show', [
'users' => fn () => User::all(),
'chatMessages' => Inertia::lazy(fn () => ChatMessages::all()),
]);
}
}
So in this example, we have a ChatController
with a show
method. The show
method returns an Inertia response with
a list of users and chat messages. The users
data is returned directly, while the chatMessages
data is returned
using the Inertia::lazy()
method. So on the initial page load, only the users data will be loaded, and the chat
messages will be loaded later when they are needed.
In the frontend you can request a partial reload like this:
Inertia.reload({component: 'Chat/Show', data: 'chatMessages'});
This will send a XHR request to the backend with the necessary headers to reload the chat messages.
Testing partial reloads
After exploring the functionality of Inertia.js partial reloads, our next step involves testing them within Laravel applications. Despite Inertia lacking dedicated testing helpers for simulating partial reloads, we can effectively test this feature by manually setting the necessary HTTP headers in our test requests. To simulate a partial reload, a test request must include the X-Inertia-Partial-Component and X-Inertia-Partial-Data headers, which specify the component and data to be reloaded, respectively. Following the request, we then verify that the response accurately reflects the expected updated data.
$this->get(route('chat.show'),
[
'X-Inertia-Partial-Component' => 'Chat/Show',
'X-Inertia-Partial-Data' => 'chatMessages',
]
)
->assertOk()
->assertInertia(fn (AssertableInertia $page) => $page
...
);
Just as we test routes without partial data using Laravel's get()
method, we apply the same approach to simulate a
request
for partial reloads. To achieve this, headers are provided as the second parameter to the ->get()
helper. The
X-Inertia-Partial-Component
header indicates the component that should be refreshed, and the X-Inertia-Partial-Data
header
specifies the data to be reloaded, such as chatMessages in our example.
Following this setup, you can perform your usual assertions. But keep in mind, that the response will only contain data you requested.
Final thoughts
Partial reloads can significantly enhance the user experience and performance of your Laravel applications by allowing specific sections of a page to update without a querying all of the data.
To implement this feature effectively, start by identifying the parts of your application that are updated frequently. These are prime candidates for partial reloads, as they benefit the most from the improved responsiveness and reduced load times.
Additionally, consider using Inertia::lazy()
for components that load data-intensive resources. This method optimizes
initial load times by deferring the loading of heavy data until it's needed, which is particularly useful for improving
the overall performance of your application.
Comprehensive testing plays a crucial role in ensuring the reliability of partial reloads. By thoroughly testing the functionality across your application, you can identify and address any potential issues, ensuring a smooth and responsive experience for users. Integrating Inertia.js partial reloads effectively requires a thoughtful approach to both development and testing.
By focusing on dynamically updated sections, optimizing data loading, and conducting rigorous testing, you can significantly enhance both the performance and user experience of your Laravel applications.
Do you need help with your next project?
Unleash the full potential of your business, and schedule a no-obligation consultation with our team of experts now!